Refunding a Guest Payment
How to refund a guest payment.
Overview

Guest Refund Flow
This document explains how to identify the payment to refund and how to process this refund.
Step 1: Retrieve the Payment ID
Available Endpoints
Method | Endpoint |
---|---|
GET | /reservations/{id} |
Key Parameters
Path Parameter | Type | Description | Required |
---|---|---|---|
id | string | Reservation ID. | ✔️ |
Query Parameter | Type | Description | Required |
---|---|---|---|
fields=money.payments | [string] | Request the payments field in the response. Narrows the data in the API response to essential fields and payments only. | Recommended |
Request
curl 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0?fields=money.payments'
var requestOptions = {
method: 'GET',
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0?fields=money.payments", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0?fields=money.payments',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = ''
headers = {}
conn.request("GET", "/v1/reservations/64da254383ad1500284bd0c0?fields=money.payments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "64da254383ad1500284bd0c0",
"integration": {
"_id": "62a72ab7c147f0003487b854",
"platform": "manual",
"limitations": {
"availableStatuses": []
}
},
"listingId": "64da223eb3de7a002a462fcc",
"guestId": "64da2084867c12ee0c2ca47f",
"accountId": "5213a2d206112710005d96ff",
"money": {
"currency": "USD",
"payments": [
{
"paymentMethodStatus": "ACTIVE",
"isAuthorizationHold": false,
"status": "PENDING",
"_id": "64da25d683ad1500284bd0ef",
"chargebacksData": {
"chargebacks": []
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"guestId": "64da2084867c12ee0c2ca47f",
"currency": "USD",
"attempts": [],
"refunds": [],
"authorizationHoldCaptures": [],
"createdAt": "2023-08-14T13:02:14.058Z",
"receiptTargets": []
},
{
"paymentMethodStatus": "ACTIVE",
"isAuthorizationHold": false,
"status": "SUCCEEDED",
"_id": "64da267c83ad1500284bd104",
"chargebacksData": {
"chargebacks": []
},
"amount": 500,
"note": "Deposit",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"guestId": "64da2084867c12ee0c2ca47f",
"currency": "USD",
"attempts": [
{
"_id": "64da267d83ad1500284bd109",
"status": "SUCCEEDED",
"payload": {
"id": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"object": "payment_intent",
"amount": 50000,
"amount_capturable": 0,
"amount_received": 50000,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee_amount": 200,
"automatic_payment_methods": null,
"canceled_at": null,
"cancellation_reason": null,
"capture_method": "automatic",
"charges": {
"object": "list",
"data": [
{
"id": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"object": "charge",
"amount": 50000,
"amount_captured": 50000,
"amount_refunded": 0,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee": "fee_1Nf0S9GfrrmRCKB1dolQDBpR",
"application_fee_amount": 200,
"balance_transaction": "txn_3Nf0S8GfrrmRCKB11uWFFji9",
"billing_details": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"email": null,
"name": "John Smith",
"phone": null
},
"calculated_statement_descriptor": "GUESTY BOOKING",
"captured": true,
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"destination": null,
"dispute": null,
"disputed": false,
"failure_balance_transaction": null,
"failure_code": null,
"failure_message": null,
"invoice": null,
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"on_behalf_of": null,
"order": null,
"outcome": {
"network_status": "approved_by_network",
"reason": null,
"risk_level": "normal",
"risk_score": 6,
"seller_message": "Payment complete.",
"type": "authorized"
},
"paid": true,
"payment_intent": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_details": {
"card": {
"brand": "visa",
"checks": {
"address_line1_check": "pass",
"address_postal_code_check": "pass",
"cvc_check": "pass"
},
"country": "US",
"ds_transaction_id": null,
"exp_month": 12,
"exp_year": 2026,
"fingerprint": "6i2ad2EHQpQJ8PeH",
"funding": "credit",
"installments": null,
"last4": "4242",
"mandate": null,
"moto": null,
"network": "visa",
"network_token": {
"used": false
},
"three_d_secure": null,
"wallet": null
},
"type": "card"
},
"receipt_email": null,
"receipt_number": null,
"receipt_url": "https://pay.stripe.com/receipts/payment/CAcaFwoVYWNjdF8xS2ZnTjRHZnJybVJDS0IxKP3M6KYGMgZvQW4a3jo6LBbT8xvMIgN5WV4QbH4rgdJw1K66qhPQKUU-PeqLw5GaFO_FgOJelXWlJsNI",
"refunded": false,
"refunds": {
"object": "list",
"data": [],
"has_more": false,
"total_count": 0,
"url": "/v1/charges/ch_3Nf0S8GfrrmRCKB1160cJBx4/refunds"
},
"review": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"source_transfer": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
}
],
"has_more": false,
"total_count": 1,
"url": "/v1/charges?payment_intent=pi_3Nf0S8GfrrmRCKB11SVF4dCI"
},
"client_secret": "pi_3Nf0S8GfrrmRCKB11SVF4dCI_secret_xrh2J2yBNN3IDaOcVQK2BwxZv",
"confirmation_method": "manual",
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"invoice": null,
"last_payment_error": null,
"latest_charge": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"next_action": null,
"on_behalf_of": null,
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_options": {
"card": {
"installments": null,
"mandate_options": null,
"network": null,
"request_three_d_secure": "automatic"
}
},
"payment_method_types": [
"card"
],
"processing": null,
"receipt_email": null,
"review": null,
"setup_future_usage": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
},
"createdAt": "2023-08-14T13:05:01.910Z"
}
],
"shouldBePaidAt": "2023-08-14T13:05:00.263Z",
"paidAt": "2023-08-14T13:05:01.820Z",
"confirmationCode": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"refunds": [
{
"_id": "64da2c0d83ad1500284bd128",
"amount": 250,
"note": "note",
"payload": {
"id": "re_3Nf0S8GfrrmRCKB11JZsNCfT",
"object": "refund",
"amount": 25000,
"balance_transaction": "txn_3Nf0S8GfrrmRCKB112KgYIx5",
"charge": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"created": 1692019724,
"currency": "usd",
"payment_intent": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"reason": "requested_by_customer",
"receipt_number": null,
"source_transfer_reversal": null,
"status": "succeeded",
"transfer_reversal": null
},
"status": "SUCCEEDED",
"createdAt": "2023-08-14T13:28:45.582Z"
}
],
"authorizationHoldCaptures": [],
"createdAt": "2023-08-14T13:05:01.910Z",
"receiptTargets": [],
"receiptId": 18801740
}
]
},
"listing": {
"_id": "64da223eb3de7a002a462fcc"
},
"guest": {
"_id": "64da2084867c12ee0c2ca47f"
}
}
The ID for the relevant payment can be found in the _id
field of the payment object under the status
field.
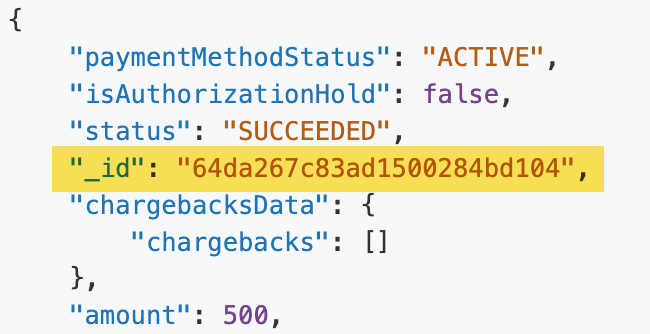
Payment Object ID
Step 2: Refund the Payment
With this request, you have the capability to process both partial and full refunds.
Available Endpoints
Method | Endpoint |
---|---|
POST | /reservations/{id}/payments/{paymentId}/refund |
Key Parameters
Path Parameter | Type | Description | Required |
---|---|---|---|
id | string | Reservation ID. | ✔️ |
paymentId | string | ID of the specific payment to be refunded. | ✔️ |
Body Parameter | Type | Description | Required |
---|---|---|---|
amount | number | The portion of the payment to refund. | ✔️ |
note | string | Add context to the refund by adding a description. |
Request
curl 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments/64da267c83ad1500284bd104/refund' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"amount": 250,
"note": "note"
}
'
var myHeaders = new Headers();
myHeaders.append("accept", "application/json");
myHeaders.append("content-type", "application/json");
var raw = JSON.stringify({
"amount": 250,
"note": "note"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments/64da267c83ad1500284bd104/refund", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments/64da267c83ad1500284bd104/refund',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"amount": 250,
"note": "note"
}
',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"amount": 250,
"note": "note"
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/reservations/64da254383ad1500284bd0c0/payments/64da267c83ad1500284bd104/refund", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "64da254383ad1500284bd0c0",
"money": {
"payments": [
{
"paymentMethodStatus": "ACTIVE",
"isAuthorizationHold": false,
"status": "SUCCEEDED",
"_id": "64da267c83ad1500284bd104",
"chargebacksData": {
"chargebacks": []
},
"amount": 500,
"note": "Deposit",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"guestId": "64da2084867c12ee0c2ca47f",
"currency": "USD",
"attempts": [
{
"_id": "64da267d83ad1500284bd109",
"status": "SUCCEEDED",
"payload": {
"id": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"object": "payment_intent",
"amount": 50000,
"amount_capturable": 0,
"amount_received": 50000,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee_amount": 200,
"automatic_payment_methods": null,
"canceled_at": null,
"cancellation_reason": null,
"capture_method": "automatic",
"charges": {
"object": "list",
"data": [
{
"id": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"object": "charge",
"amount": 50000,
"amount_captured": 50000,
"amount_refunded": 0,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee": "fee_1Nf0S9GfrrmRCKB1dolQDBpR",
"application_fee_amount": 200,
"balance_transaction": "txn_3Nf0S8GfrrmRCKB11uWFFji9",
"billing_details": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"email": null,
"name": "John Smith",
"phone": null
},
"calculated_statement_descriptor": "GUESTY BOOKING",
"captured": true,
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"destination": null,
"dispute": null,
"disputed": false,
"failure_balance_transaction": null,
"failure_code": null,
"failure_message": null,
"invoice": null,
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"on_behalf_of": null,
"order": null,
"outcome": {
"network_status": "approved_by_network",
"reason": null,
"risk_level": "normal",
"risk_score": 6,
"seller_message": "Payment complete.",
"type": "authorized"
},
"paid": true,
"payment_intent": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_details": {
"card": {
"brand": "visa",
"checks": {
"address_line1_check": "pass",
"address_postal_code_check": "pass",
"cvc_check": "pass"
},
"country": "US",
"ds_transaction_id": null,
"exp_month": 12,
"exp_year": 2026,
"fingerprint": "6i2ad2EHQpQJ8PeH",
"funding": "credit",
"installments": null,
"last4": "4242",
"mandate": null,
"moto": null,
"network": "visa",
"network_token": {
"used": false
},
"three_d_secure": null,
"wallet": null
},
"type": "card"
},
"receipt_email": null,
"receipt_number": null,
"receipt_url": "https://pay.stripe.com/receipts/payment/CAcaFwoVYWNjdF8xS2ZnTjRHZnJybVJDS0IxKP3M6KYGMgZvQW4a3jo6LBbT8xvMIgN5WV4QbH4rgdJw1K66qhPQKUU-PeqLw5GaFO_FgOJelXWlJsNI",
"refunded": false,
"refunds": {
"object": "list",
"data": [],
"has_more": false,
"total_count": 0,
"url": "/v1/charges/ch_3Nf0S8GfrrmRCKB1160cJBx4/refunds"
},
"review": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"source_transfer": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
}
],
"has_more": false,
"total_count": 1,
"url": "/v1/charges?payment_intent=pi_3Nf0S8GfrrmRCKB11SVF4dCI"
},
"client_secret": "pi_3Nf0S8GfrrmRCKB11SVF4dCI_secret_xrh2J2yBNN3IDaOcVQK2BwxZv",
"confirmation_method": "manual",
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"invoice": null,
"last_payment_error": null,
"latest_charge": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"next_action": null,
"on_behalf_of": null,
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_options": {
"card": {
"installments": null,
"mandate_options": null,
"network": null,
"request_three_d_secure": "automatic"
}
},
"payment_method_types": [
"card"
],
"processing": null,
"receipt_email": null,
"review": null,
"setup_future_usage": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
},
"createdAt": "2023-08-14T13:05:01.910Z"
}
],
"shouldBePaidAt": "2023-08-14T13:05:00.263Z",
"paidAt": "2023-08-14T13:05:01.820Z",
"confirmationCode": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"refunds": [
{
"_id": "64da2c0d83ad1500284bd128",
"amount": 250,
"note": "note",
"payload": {
"id": "re_3Nf0S8GfrrmRCKB11JZsNCfT",
"object": "refund",
"amount": 25000,
"balance_transaction": "txn_3Nf0S8GfrrmRCKB112KgYIx5",
"charge": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"created": 1692019724,
"currency": "usd",
"payment_intent": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"reason": "requested_by_customer",
"receipt_number": null,
"source_transfer_reversal": null,
"status": "succeeded",
"transfer_reversal": null
},
"status": "SUCCEEDED",
"createdAt": "2023-08-14T13:28:45.582Z"
}
],
"authorizationHoldCaptures": [],
"createdAt": "2023-08-14T13:05:01.910Z",
"receiptTargets": [],
"receiptId": 18801740,
"guest": {
"_id": "64da2084867c12ee0c2ca47f",
"email": "[email protected]",
"fullName": "Irving Washington"
},
"paymentMethod": {
"_id": "64da2367d8d9771c02befbb2",
"method": "STRIPE",
"last4": "4242",
"brand": "visa"
}
}
]
}
}
Updated 12 months ago