Query Available Listings
Retrieving available listings via the OAPI
Read First
The best resource for querying available listings is the newer Booking Engine API's listings endpoint. It has more features. Only if you have an existing integration built on our first generation Open API (OAPI) booking flow should you rely on this guide.
Key Endpoints
Method | Endpoint |
---|---|
GET | /listings |
Searching for Availability
The availability query returns listings that meet the parameters specified. A listing won't be included in the response if it is unavailable.
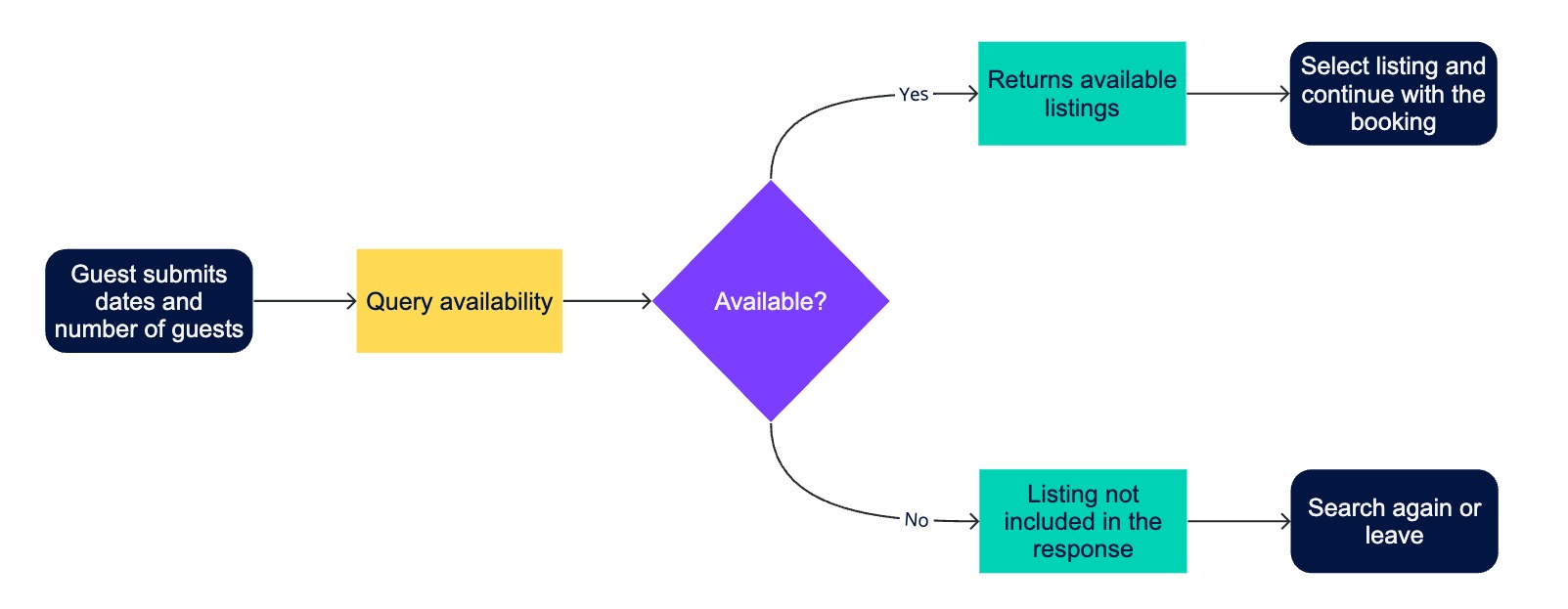
Querying Available Listings Flow
Key Parameters
Several query parameters can be employed when searching for available listings for a defined period of stay.
Query Parameter | Type | Description | Required |
---|---|---|---|
ids | string | Search specific listings for availability. | |
nids | string | Exclude specific listings from the query and response. | |
available | object | For defining the dates and minimum stay. E.g. available={"checkIn":"YYYY-MM-DD","checkOut":"YYYY-MM-DD","minOccupancy":3} | ✔️ |
city | string | Filter by the city in which the listing is located. | |
tags | string | Filter by listing tags. | |
ignoreFlexibleBlocks | boolean | Ignore manual, advance notice, and preparation time blocks. |
Examples
Querying Availability at Multiple Listings
The following is a query for available listings in a specific city with a minimum occupancy of two. To return specific listing fields in your response, include them in your query. This example includes the listing ID, nickname, title, type, address, terms, and prices.
Request
curl --globoff 'https://open-api.guesty.com/v1/listings?city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available={%22checkIn%22%3A%222023-09-01%22%2C%22checkOut%22%3A%222023-09-10%22%2C%22minOccupancy%22%3A2}' \
--header 'Accept: application/json' \
--header 'authorization: {accessToken}'
var myHeaders = new Headers();
myHeaders.append("Accept", "application/json");
myHeaders.append("authorization", "{accessToken}");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/listings?city=Sydney&fields=_id nickname title type address terms prices&available={\"checkIn\":\"2023-09-01\",\"checkOut\":\"2023-09-10\",\"minOccupancy\":2}", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/listings?city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available={%22checkIn%22%3A%222023-09-01%22%2C%22checkOut%22%3A%222023-09-10%22%2C%22minOccupancy%22%3A2}',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Accept: application/json',
'authorization: {accessToken}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = ''
headers = {
'Accept': 'application/json',
'authorization': '{accessToken}'
}
conn.request("GET", "/v1/listings?city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available=%7B%22checkIn%22:%222023-09-01%22,%22checkOut%22:%222023-09-10%22,%22minOccupancy%22:2%7D", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"results": [
{
"_id": "5e1c3bec4ffe9100218e3e0d",
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "ILS",
"basePrice": 100,
"cleaningFee": 20,
"guestsIncludedInRegularFee": 1,
"extraPersonFee": 20
},
"type": "MTL_CHILD",
"tags": [],
"address": {
"full": "110-140 King St, Sydney NSW 2000, Australia",
"lng": 151.20931,
"lat": -33.8688222,
"street": "King Street 110-140",
"city": "Sydney",
"country": "Australia",
"state": "New South Wales",
"zipcode": "2000",
"county": null
},
"nickname": "DemoListingNick",
"title": "DemoListingTitle",
"accountId": "5b852676354b34003f0a55eb"
},
{
"_id": "6034db72fc09e3002cf64bdf",
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "ILS",
"basePrice": 100,
"cleaningFee": 20,
"guestsIncludedInRegularFee": 1,
"extraPersonFee": 20
},
"type": "MTL",
"tags": [],
"address": {
"full": "110 King St, Sydney NSW 2000, Australia",
"lng": 151.2092013,
"lat": -33.869002,
"street": "King Street 110",
"city": "Sydney",
"country": "Australia",
"state": "New South Wales",
"zipcode": "2000",
"county": null
},
"nickname": "DemoListingNick (1)",
"title": "DemoListingTitle",
"accountId": "5b852676354b34003f0a55eb"
},
{
"_id": "62ec6975134d780032b62221",
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "AUD",
"basePrice": 100
},
"type": "SINGLE",
"tags": [
"shan"
],
"address": {
"full": "77 King St, Sydney NSW 2000, Australia",
"lng": 151.2066116,
"lat": -33.8689358,
"street": "King Street 77",
"city": "Sydney",
"country": "Australia",
"zipcode": "2000",
"state": "New South Wales"
},
"nickname": "Test_Listing_Shan",
"title": "Test Listing Shans",
"accountId": "5b852676354b34003f0a55eb"
}
],
"title": "Listings Report",
"fields": "_id nickname title type address terms prices",
"limit": 25,
"skip": 0,
"count": 3
}
Querying Availability at a Specific Listing
In this scenario, a guest has narrowed down their choice of listings and wishes to know whether they're available for a range of dates.
Request
curl --globoff 'https://open-api.guesty.com/v1/listings?ids=62ec6975134d780032b62221&city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available={%22checkIn%22%3A%222023-09-01%22%2C%22checkOut%22%3A%222023-09-10%22%2C%22minOccupancy%22%3A2}' \
--header 'Accept: application/json' \
--header 'authorization: {accessToken}'
var myHeaders = new Headers();
myHeaders.append("Accept", "application/json");
myHeaders.append("authorization", "{accessToken}");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/listings?ids=62ec6975134d780032b62221&city=Sydney&fields=_id nickname title type address terms prices&available={\"checkIn\":\"2023-09-01\",\"checkOut\":\"2023-09-10\",\"minOccupancy\":2}", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/listings?ids=62ec6975134d780032b62221&city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available={%22checkIn%22%3A%222023-09-01%22%2C%22checkOut%22%3A%222023-09-10%22%2C%22minOccupancy%22%3A2}',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Accept: application/json',
'authorization: {accessToken}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = ''
headers = {
'Accept': 'application/json',
'authorization': '{accessToken}'
}
conn.request("GET", "/v1/listings?ids=62ec6975134d780032b62221&city=Sydney&fields=_id%20nickname%20title%20type%20address%20terms%20prices&available=%7B%22checkIn%22:%222023-09-01%22,%22checkOut%22:%222023-09-10%22,%22minOccupancy%22:2%7D", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"results": [
{
"_id": "62ec6975134d780032b62221",
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "AUD",
"basePrice": 100
},
"type": "SINGLE",
"tags": [
"shan"
],
"address": {
"full": "77 King St, Sydney NSW 2000, Australia",
"lng": 151.2066116,
"lat": -33.8689358,
"street": "King Street 77",
"city": "Sydney",
"country": "Australia",
"zipcode": "2000",
"state": "New South Wales"
},
"nickname": "Test_Listing_Shan",
"title": "Test Listing Shans",
"accountId": "5b852676354b34003f0a55eb"
}
],
"title": "Listings Report",
"fields": "_id nickname title type address terms prices",
"limit": 25,
"skip": 0,
"count": 1
}
Updated 11 months ago