Authentication
Generating an access token to authenticate Open API requests.
Proper Authentication
Open API (OAPI) access keys are for authenticating OAPI requests only. To authenticate requests to our Booking Engine API, please follow its authentication steps here.
1. Creating Authorization Keys
Guesty's new Open API is a REST API that uses OAuth 2.0 access tokens to authenticate requests. Your access token authorizes you to use the Guesty Open API server and can be reused until it expires.
You must exchange your Client ID and Client Secret for an access token to authenticate your requests. You can create these keys by logging into your Guesty account and following these instructions.
🚧 Important
- Your Client Secret is only visible the first time you access it. After that, Guesty redacts the Client
Secret for your security. Make sure to store it in a safe place where you can access it as needed.- If you're using the old Guesty open API, please follow these instructions for migration.
2. Generating the Access Token
The following examples show you how to get your access token using cURL. Please copy the following code and modify it.
curl --location --request POST 'https://open-api.guesty.com/oauth2/token' \
--header 'Accept: application/json' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'scope=open-api' \
--data-urlencode 'client_secret=<CLIENT_SECRET>' \
--data-urlencode 'client_id=<CLIENT_ID>'
curl --location --request POST 'https://open-api.guesty.com/oauth2/token' \
--user '<CLIENT_ID>:<CLIENT_SECRET>' \
--header 'Accept: application/json' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'scope=open-api'
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://open-api.guesty.com/oauth2/token',
'headers': {
'Accept': 'application/json',
'Content-Type': 'application/x-www-form-urlencoded'
},
form: {
'grant_type': 'client_credentials',
'scope': 'open-api',
'client_secret': '<CLIENT_SECRET>',
'client_id': '<CLIENT_ID>'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
var settings = {
"url": "https://open-api.guesty.com/oauth2/token",
"method": "POST",
"timeout": 0,
"headers": {
"Accept": "application/json",
"Content-Type": "application/x-www-form-urlencoded"
},
"data": {
"grant_type": "client_credentials",
"scope": "open-api",
"client_secret": "<CLIENT_SECRET>",
"client_id": "<CLIENT_ID>"
}
};
$.ajax(settings).done(function (response) {
console.log(response);
});
- Change
CLIENT SECRET
to your Client Secret. - Change
CLIENT ID
to your Client ID.
Sample Response
Guesty returns an access token, the number of seconds the access token is valid (equal to 24 hours), token type, and scope. You may reuse the token as often as you need within that period.
{
"token_type": "Bearer",
"expires_in": 86400,
"access_token": "eyJraWQiOiJydFFaWXhoTzBtNlllbWZaRnRBRXJORFVkWThZOFlPeGxndVZabmpJZVNvIiwiYWxnIjoiUlMyNTYifQ.eyJ2ZXIiOjEsImp0aSI6IkFULlVWdkZ5NW5ES1h4SlBvUVUzbWN3ZS1ORXp0eHo0NWNQVktZVUFxM3V5RXMiLCJpc3MiOiJodHRwczovL2xvZ2luLmd1ZXN0eS5jb20vb2F1dGgyL2F1czFwOHFyaDUzQ2NRVEk5NWQ3IiwiYXVkIjoiaHR0cHM6Ly9vcGVuLWFwaS5ndWVzdHkuY29tIiwiaWF0IjoxNjU5NTM3NjUwLCJleHAiOjE2NTk2MjQwNTAsImNpZCI6IjBvYTViaWw0MzB4OHpMeldCNWQ3Iiwic2NwIjpbIm9wZW4tYXBpIl0sInJlcXVlc3RlciI6IkVYVEVSTkFMIiwiYWNjb3VudElkIjoiNjJhMDZkMmYyMjUxMzAwMDM1OWVlODkzIiwic3ViIjoiMG9hNWJpbDQzMHg4ekx6V0I1ZDciLCJ1c2VyUm9sZXMiOlt7InJvbGVJZCI6eyJwZXJtaXNzaW9ucyI6WyJhZG1pbiJdfX1dLCJyb2xlIjoidXNlciIsImlhbSI6InYzIiwibmFtZSI6IkNTIHwgVEEtMSB8IE1vc2hlIn0.LlZZUhM4WTsIsgmuqLasl-5WtNx0N8MvpmSGerSz5DpvO2AkcOhZAuYgPh1xqocGpwcKLMBokYvSyC0xRtptDEpaEY8X__ozvDS_UpUp2vKdtU2t-1ns7ut5qZlGhf6ffZAR0K1WXEb1081n-0Ms5qxfy1HbWkmyPUt0tgN-xAmRgnbSX01YELZ-_vovpitsxC0JYPPpBOi_w8kxlxsqKLWiFzDe5SpzBUYncjJEafISXzo5PNHEweHkvguXXM9xVXlNpE_q0DfQvQ41mn8TDnhUVtspscG3WmKV86k5QAjqHyYMJ2_2WOWRWrjfeyKc5ePC1HqCANRxOO7oS7dQcA",
"scope": "open-api"
}
You can generate a maximum of five access tokens per 24 hours, per clientId
.
✅ Best Practices
- To minimize the chance of errors, store the value of the
expires_in
field locally, using it to ensure your token is refreshed 30 - 60 minutes before it expires.- To avoid the rate limits on the
/oauth2
endpoint, we advise calling it once a day, and caching the token for 24 hours. Use this same token for any other Open API requests within that period.- Alternatively, you can also adopt a reactive approach and choose to refresh your token after it expires. You will receive a
403 - Unauthorized
error when your token is expired. You can handle the 403 error and refresh your access token accordingly.
3. Using the Access Token
When you make a request to the Open API, include the access token in the Authorization header with the designation Bearer
.
Example
curl --location --request GET 'https://open-api.guesty.com/v1/listings' \
--header 'accept: application/json' \
--header 'Authorization: Bearer eyJraWQiOiJydFFaWXhoTzBtNlllbWZaRnRBRXJORFVkWThZOFlPeGxndVZabmpJZVNvIiwiYWxnIjoiUlMyNTYifQ.eyJ2ZXIiOjEsImp0aSI6IkFULlVWdkZ5NW5ES1h4SlBvUVUzbWN3ZS1ORXp0eHo0NWNQVktZVUFxM3V5RXMiLCJpc3MiOiJodHRwczovL2xvZ2luLmd1ZXN0eS5jb20vb2F1dGgyL2F1czFwOHFyaDUzQ2NRVEk5NWQ3IiwiYXVkIjoiaHR0cHM6Ly9vcGVuLWFwaS5ndWVzdHkuY29tIiwiaWF0IjoxNjU5NTM3NjUwLCJleHAiOjE2NTk2MjQwNTAsImNpZCI6IjBvYTViaWw0MzB4OHpMeldCNWQ3Iiwic2NwIjpbIm9wZW4tYXBpIl0sInJlcXVlc3RlciI6IkVYVEVSTkFMIiwiYWNjb3VudElkIjoiNjJhMDZkMmYyMjUxMzAwMDM1OWVlODkzIiwic3ViIjoiMG9hNWJpbDQzMHg4ekx6V0I1ZDciLCJ1c2VyUm9sZXMiOlt7InJvbGVJZCI6eyJwZXJtaXNzaW9ucyI6WyJhZG1pbiJdfX1dLCJyb2xlIjoidXNlciIsImlhbSI6InYzIiwibmFtZSI6IkNTIHwgVEEtMSB8IE1vc2hlIn0.LlZZUhM4WTsIsgmuqLasl-5WtNx0N8MvpmSGerSz5DpvO2AkcOhZAuYgPh1xqocGpwcKLMBokYvSyC0xRtptDEpaEY8X__ozvDS_UpUp2vKdtU2t-1ns7ut5qZlGhf6ffZAR0K1WXEb1081n-0Ms5qxfy1HbWkmyPUt0tgN-xAmRgnbSX01YELZ-_vovpitsxC0JYPPpBOi_w8kxlxsqKLWiFzDe5SpzBUYncjJEafISXzo5PNHEweHkvguXXM9xVXlNpE_q0DfQvQ41mn8TDnhUVtspscG3WmKV86k5QAjqHyYMJ2_2WOWRWrjfeyKc5ePC1HqCANRxOO7oS7dQcA'
When your access token expires, repeat the /oauth2/token
request to retrieve a new access token.
Expired Token
You'll know your token has expired when you receive a status 403 error with the following message:
{ "message": "You don't have permission to access, please contact Guesty support." }
Using Postman to Generate an Access Token
Step-by-Step
In the Postman app, complete the following:
- Set the request method to POST.
- Enter
https://open-api.guesty.com/oauth2/token
as the request URL.

Figure 1: Method and URL
- Select the Authorization tab.
- From the TYPE list, select Basic Auth.
- In the Username field, enter your Client ID.
- In the Password field, enter your Client Secret.
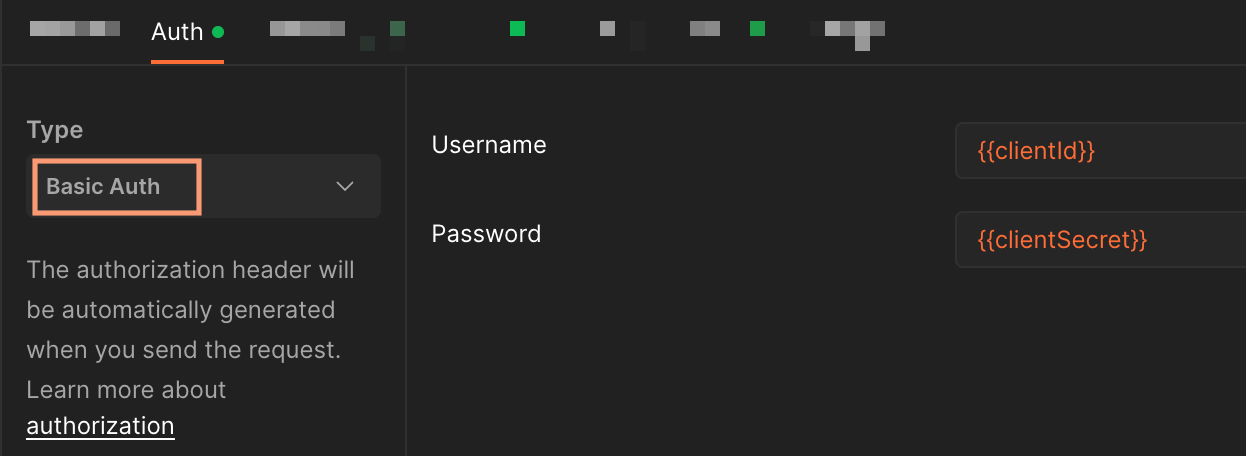
Figure 2: Authentication
- Select the Body tab.
- Select the x-www-form-urlencoded option.
- In the KEY field, enter
grant_type
. - In the VALUE field, enter
client_credentials
. - In the second KEY field, enter
scope
. - In the VALUE field, enter
open-api
.
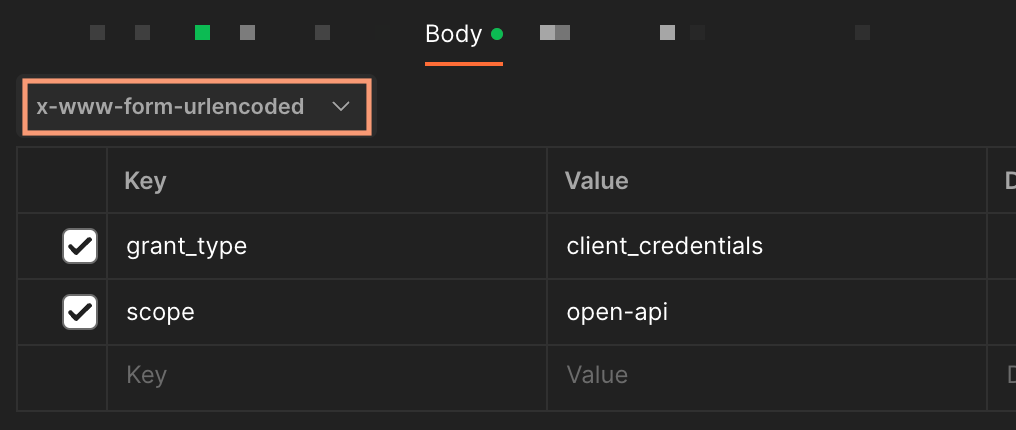
Figure 3: Body
- Click Send.
Expected Response
Status 200 OK
{
"token_type": "Bearer",
"expires_in": 86400,
"access_token": "<accessToken>",
"scope": "open-api"
}
Updated 4 months ago