Create a Guest and Payment Method
Learn how to create a guest and link a payment method for processing reservation payments.
Overview
This document explains how to create a guest and attach their payment method in preparation for creating a reservation for them.
Available Endpoints
Method | Endpoint |
---|---|
POST | /guests-crud |
POST | /guests/{id}/payment-methods |
Create a New Guest Profile
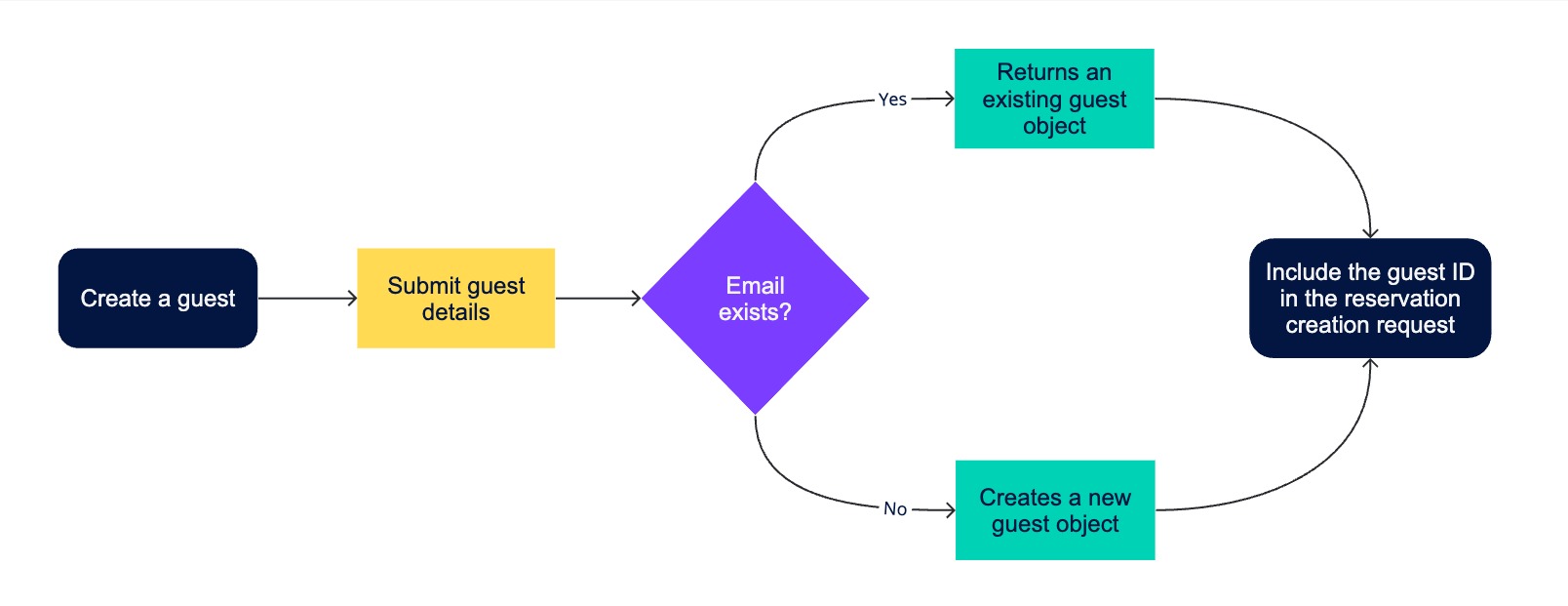
Guest Creation Flow
Returning Guests
Guesty automatically reconciles new submissions with existing data by matching the supplied email to an existing guest record on your account. If a match is found, new details are added to the existing record. If not, a new guest record is created.
Key Parameters
Body Parameter | Type | Description | Required |
---|---|---|---|
firstName | string | Guest's given name. | ✔️ |
lastName | string | Guest's surname. | ✔️ |
email | string | Guest's primary email. | |
emails | [string] | All the guest's email addresses, Primary and secondary. | |
phone | string | Guest's primary phone number. | |
phones | [string] | All the guest's phone numbers, primary and secondary. | |
hometown | string | Origin of the guest. | |
address | object | Guest's primary or postal address. | |
picture | object | A link to the guest's picture(s). | |
pronouns | string | How the guest prefers to be referred in the third person. |
A complete list of parameters can be found on the Create guest reference document. We recommend including the guest's email and phone number as standard practice.
Example
curl 'https://open-api.guesty.com/v1/guests-crud' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data-raw '
{
"firstName": "Irving",
"lastName": "Washington",
"hometown": "New York",
"email": "[email protected]",
"phone": "+12128765234",
"nationality": "us",
"pronouns": "he/him/his",
"gender": "male",
"preferredLanguage": "en"
}
'
var settings = {
"url": "https://open-api.guesty.com/v1/guests-crud",
"method": "POST",
"timeout": 0,
"headers": {
"accept": "application/json",
"content-type": "application/json"
},
"data": JSON.stringify({
"firstName": "Irving",
"lastName": "Washington",
"hometown": "New York",
"email": "[email protected]",
"phone": "+12128765234",
"nationality": "us",
"pronouns": "he/him/his",
"gender": "male",
"preferredLanguage": "en"
}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/guests-crud',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"firstName": "Irving",
"lastName": "Washington",
"hometown": "New York",
"email": "[email protected]",
"phone": "+12128765234",
"nationality": "us",
"pronouns": "he/him/his",
"gender": "male",
"preferredLanguage": "en"
}
',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"firstName": "Irving",
"lastName": "Washington",
"hometown": "New York",
"email": "[email protected]",
"phone": "+12128765234",
"nationality": "us",
"pronouns": "he/him/his",
"gender": "male",
"preferredLanguage": "en"
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/guests-crud", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"createdAt": "2023-07-31T12:43:33.218Z",
"paymentProvidersCustomers": [],
"paymentMethodIds": [],
"communicationMethods": [
"email",
"sms"
],
"nationality": "us",
"isOtaModified": false,
"otaLinks": [],
"pronouns": "he/him/his",
"interests": [],
"allergies": [],
"dietaryPreferences": [],
"gender": "male",
"preferredLanguage": "en",
"tags": [],
"airbnb2": {
"id": "1180340821319"
},
"airbnb": {
"id": "1180340812902"
},
"phone": "12128765234",
"phones": [
"12128765234"
],
"email": "[email protected]",
"emails": [
"[email protected]"
],
"verifications": [],
"hometown": "New York",
"fullName": "Irving Washington",
"lastName": "Washington",
"firstName": "Irving",
"accountId": "5b852676354b34003f0a55eb",
"_id": "64c7afe8a1237cba15116c95",
"pictures": [],
"__v": 0
}
Take note of the _id
in the response. You will use it to assign the guest to the reservation you create. If the guest is a returning guest, the ID in the response will be that of the existing record.
Guest Payment Methods

Guest Payment Method Flow
Relationship with Reservations
The creation and confirmation of a reservation isn't dependent on the validity of the payment method. It will be processed regardless of the payment method's status.
Guesty integrates with several popular payment processors. Details can be found in our Help Center. When using any of these payment processors, you can attach a payment method token that Guesty can use to collect payments off the reservation. Learn how to tokenize the payment method here.
Key Parameters
This path parameter is required for posting all supported payment methods.
Path Parameter | Type | Description | Required |
---|---|---|---|
id | string | Guest ID | ✔️ |
Examples
All Supported Payment Processors
This corresponds to OPTION 2 on the reference document.
Key Parameters
Body Parameter | Type | Description | Required |
---|---|---|---|
_id | string | The ID from the GuestyPay tokenization response. | ✔️ |
paymentProviderId | string | Guesty ID for the GuestyPay payment method. See Payment Methods. | ✔️ |
reservationId | string | Specifies the use of this payment method with a specific booking. |
Request
curl --request POST \
--url https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods \
--header 'content-type: application/json' \
--data '
{
"_id": "849c9df2-a326-4cf6-9106-ded9b893d7e6",
"paymentProviderId": "646f0a20bffea29d7adc0d53",
"reservationId": "563e0b6a08a2710e00057b82"
}
'
var settings = {
"url": "https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods",
"method": "POST",
"timeout": 0,
"headers": {
"content-type": "application/json",
"Authorization": "<accessToken>"
},
"data": JSON.stringify({
"_id": "6265d1b6a08a2710e00057b82",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b",
"reservationId": "563e0b6a08a2710e00057b82"
}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"_id": "6265d1b6a08a2710e00057b82",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b",
"reservationId": "563e0b6a08a2710e00057b82"
}
',
CURLOPT_HTTPHEADER => array(
'content-type: application/json',
'Authorization: Bearer <accessToken>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"_id": "6265d1b6a08a2710e00057b82",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b",
"reservationId": "563e0b6a08a2710e00057b82"
})
headers = {
'content-type': 'application/json',
'Authorization': 'Bearer <accessToken>'
}
conn.request("POST", "/v1/guests/64c7afe8a1237cba15116c95/payment-methods", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "64d2001a85b0ffa9d8e48127",
"accountId": "5213a2d206112710005d96ff",
"paymentProviderId": "646f0a20bffea29d7adc0d53",
"method": "AMARYLLIS",
"payload": {
"PaymentToken": "849c9df2-a326-4cf6-9106-ded9b893d7e6",
"TransactionId": "1387001227256341906",
"MerchantTransactionId": "Reservation-2000363",
"ResponseCode": 0,
"ResponseDescription": "OK",
"ProcessorResult": {
"TransactionId": "pay_ajkwmd2kq2nuth5u5ml2jxnu7a",
"AuthNumber": "559035",
"ResponseCode": 10000,
"ResponseDescription": "Approved",
"CvvResult": null,
"AvsResult": null
},
"billing_details": {
"name": "John Smith",
"address": {
"line1": "20 W 34th St",
"city": "New York",
"postal_code": "10001",
"country": "GB",
"state": "NA"
},
"cardHolderIdNumber": "123456789"
},
"card": {
"last4": "4242",
"exp_month": 12,
"exp_year": 2024,
"brand": "Visa"
},
"id": "849c9df2-a326-4cf6-9106-ded9b893d7e6",
"tokenId": "849c9df2-a326-4cf6-9106-ded9b893d7e6"
},
"saveForFutureUse": true,
"createdAt": "2023-08-08T08:43:08.728Z",
"last4": "4242",
"brand": "Visa",
"cardHolderIdNumber": "123456789",
"status": "ACTIVE",
"type": "PHYSICAL",
"updatedAt": "2023-08-08T08:43:06.242Z",
"__v": 0,
"guestId": "6485a5ba33533b002681d64f"
}
Payload
The payload may vary according to the card and payment processor.
External Stripe Token
This corresponds to OPTION 1 on the reference document.
Key Parameters
Body Parameter | Type | Description | Required |
---|---|---|---|
stripeCardToken | string | Stripe payment method. E.g pm_1NZw6s2eZvKYlo2CgGse32xR | ✔️ |
paymentProviderId | string | Guesty ID for the Stripe payment method. See Payment Methods. | ✔️ |
reservationId | string | Specifies the use of this payment method with a specific booking. | |
skipSetupIntent | string | true if the credit card was collected with setup_intent performed on the frontend. See Set up future payments. |
Request
curl --request POST \
--url https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods \
--header 'content-type: application/json' \
--data '
{
"stripeCardToken": "pm_1NZw6s2eZvKYlo2CgGse32xR",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b"
}
'
var settings = {
"url": "https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods",
"method": "POST",
"timeout": 0,
"headers": {
"content-type": "application/json",
"Authorization": "Bearer <accessToken>"
},
"data": JSON.stringify({
"stripeCardToken": "pm_1NZw6s2eZvKYlo2CgGse32xR",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b"
}),
};
$.ajax(settings).done(function (response) {
console.log(response);
});
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/guests/64c7afe8a1237cba15116c95/payment-methods',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"stripeCardToken": "pm_1NZw6s2eZvKYlo2CgGse32xR",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b"
}
',
CURLOPT_HTTPHEADER => array(
'content-type: application/json',
'Authorization: Bearer <accessToken>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"stripeCardToken": "pm_1NZw6s2eZvKYlo2CgGse32xR",
"paymentProviderId": "5fe4b21675087f01a3c5ab5b"
})
headers = {
'content-type': 'application/json',
'Authorization': 'Bearer <accessToken>'
}
conn.request("POST", "/v1/guests/64c7afe8a1237cba15116c95/payment-methods", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"accountId": "5213a2d206112710005d96ff",
"guestId": "637fa060f4b0d10037db4e00",
"paymentProviderId": "5f79927568c585002cc14f24",
"method": "STRIPE",
"payload": {
"id": "pm_1LwT2ILYcJ0oiNhYK4HnO4nl",
"object": "payment_method",
"billing_details": {
"address": {
"city": "test",
"country": "IL",
"line1": "kaplan",
"line2": null,
"postal_code": "90805",
"state": "tel aviv"
},
"email": null,
"name": "dan test",
"phone": null
},
"card": {
"brand": "visa",
"checks": {
"address_line1_check": "pass",
"address_postal_code_check": "pass",
"cvc_check": "pass"
},
"country": "US",
"exp_month": 4,
"exp_year": 2024,
"fingerprint": "6i2ad2EHQpQJ8PeH",
"funding": "credit",
"generated_from": null,
"last4": "4242",
"networks": {
"available": [
"visa"
],
"preferred": null
},
"three_d_secure_usage": {
"supported": true
},
"wallet": null
},
"created": 1666627079,
"customer": "cus_MfofIZCGM8jZBc",
"livemode": false,
"type": "card",
"tokenId": "pm_1LwT2ILYcJ0oiNhYK4HnO4nl"
},
"saveForFutureUse": true,
"createdAt": "2023-08-07T12:17:28.535Z",
"last4": "4242",
"brand": "visa",
"status": "ACTIVE",
"type": "PHYSICAL",
"confirmationCode": "OgJ78EwlG",
"_id": "64d0e0d885b0ffa9d8e4808c",
"updatedAt": "2023-08-07T12:17:28.536Z",
"__v": 0
}
Updated 11 months ago