Posting a Guest Payment
How to collect payment from a Guest and how to return a refund to the guest.
Overview
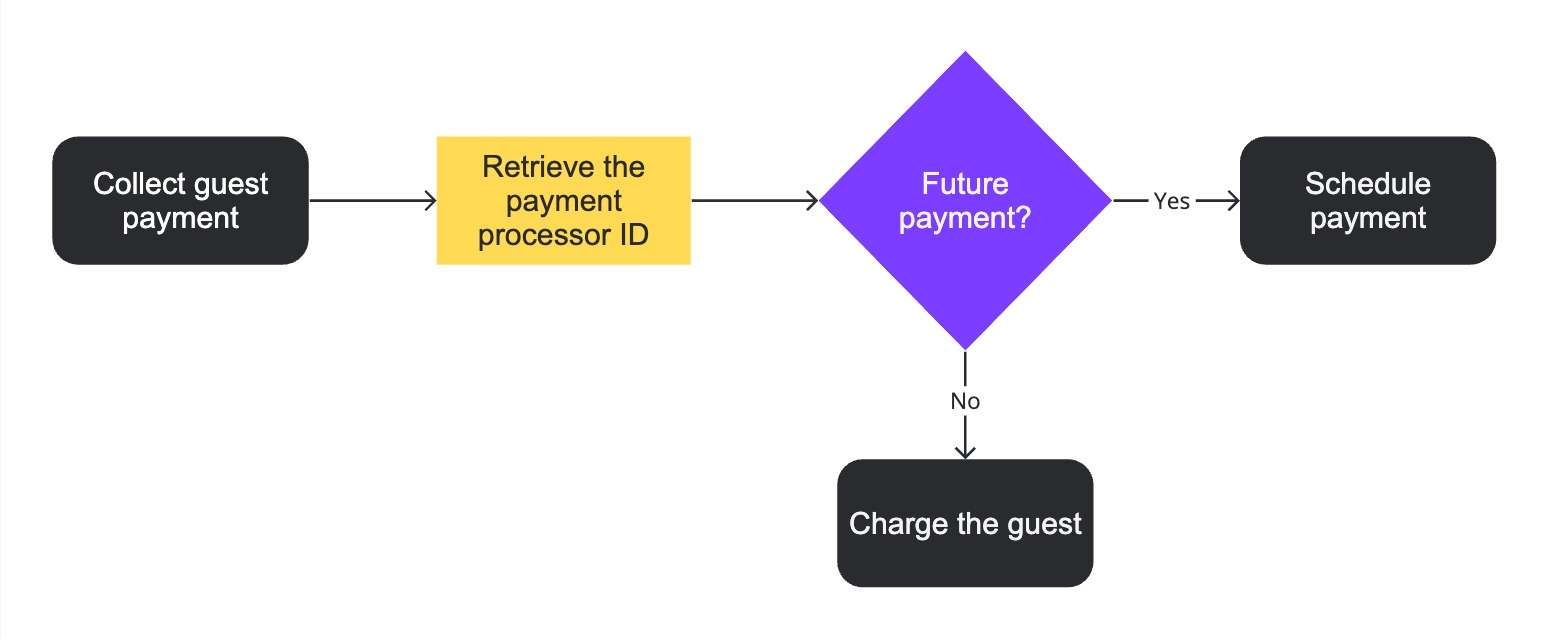
Payment Collection Flow
The payments path of the Reservations API allows you to post a payment to a reservation for immediate or future processing. This document describes posting a payment for all of Guesty's supported payment processors.
Auto Payments Deactivation
Please be aware that if you make any manual payment modifications, whether through the API or UI, the Auto Payments feature for your reservation will be turned off. This means that existing payments won't be adjusted, and no new payments will be generated to accommodate the change.
The reason for this is that the feature assumes that your manual intervention is a request to handle the reservation's financials and payments yourself, giving you complete control over the remaining payment actions in the scenario at hand. Therefore, it is recommended that you review your upcoming payments and adjust or cancel them as needed.
Available Endpoints
Method | Endpoint |
---|---|
GET | /guests/{id}/payment-methods |
POST | /reservations/{id}/payments |
Posting a Payment
Key Parameters
Path Parameter | Type | Description | Required |
---|---|---|---|
id | string | The reservation ID. | ✔️ |
Body Parameter | Type | Description | Required |
---|---|---|---|
paymentMethod | object | The payment method object. It identifies the method and payment processor. See the following table for details. | ✔️ |
amount | number | The amount to charge or refund a guest. | ✔️ |
shouldBePaidAt | date | ISO date for scheduling a future payment or refund. E.g. "2023-05-30T12:00:00.000Z" . Excluding this parameter will process the charge immediately. | |
note | string | Free text field for adding further context to the payment/refund. | |
isAuthorizationHold | boolean | For placing an authorization hold on the guest's credit card. true /false . |
The Payment Method Object
Body Parameter | Type | Description | Required |
---|---|---|---|
method | string | The name of the payment method. Select from the following table below. | ✔️ |
saveForFutureUse | boolean | Set to true if you wish to process additional charges with this specific method. Set it to false if it isn't intended to be used for additional charges. | |
id | string | The payment method ID. Select the string that matches the method attached to the guest from this call. | ✔️ |
Payment Processor Payment Method Names
Name | Description |
---|---|
STRIPE | The Stripe payment processor integration. |
AMARYLLIS | For a GuestyPay, Merchant Warrior, or Hyp payment processor integration. |
Retrieve a List of the Guest's Payment Methods
Request
curl 'https://open-api.guesty.com/v1/guests/64da2084867c12ee0c2ca47f/payment-methods' \
--header 'content-type: application/json'
var myHeaders = new Headers();
myHeaders.append("content-type", "application/json");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/guests/64da2084867c12ee0c2ca47f/payment-methods", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/guests/64da2084867c12ee0c2ca47f/payment-methods',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = ''
headers = {
'content-type': 'application/json'
}
conn.request("GET", "/v1/guests/64da2084867c12ee0c2ca47f/payment-methods", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
[
{
"_id": "64da2367d8d9771c02befbb2",
"accountId": "5213a2d206112710005d96ff",
"paymentProviderId": "623833c1486b1c0033604efc",
"method": "STRIPE",
"payload": {
// Varies depending on the card and payment processor
},
"saveForFutureUse": true,
"createdAt": "2023-08-14T12:52:05.012Z",
"last4": "4242",
"brand": "visa",
"status": "ACTIVE",
"type": "PHYSICAL",
"updatedAt": "2023-08-14T12:51:51.082Z",
"__v": 0,
"guestId": "64da2084867c12ee0c2ca47f"
}
]
Scheduling a Future Payment
Since this payment is scheduled for the future, we include the shouldBePaidAt
body parameter.
Request
curl 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment"
}
'
curl 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment"
}
'
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment"
}
',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": True,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment"
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/reservations/64da254383ad1500284bd0c0/payments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "64da254383ad1500284bd0c0",
"money": {
"payments": [
{
"paymentMethodStatus": "ACTIVE",
"isAuthorizationHold": false,
"status": "PENDING",
"_id": "64da25d683ad1500284bd0ef",
"chargebacksData": {
"chargebacks": []
},
"amount": 1200,
"shouldBePaidAt": "2023-09-01T12:00:00.000Z",
"note": "Second installment",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"guestId": "64da2084867c12ee0c2ca47f",
"currency": "USD",
"attempts": [],
"refunds": [],
"authorizationHoldCaptures": [],
"createdAt": "2023-08-14T13:02:14.058Z",
"receiptTargets": [],
"guest": {
"_id": "64da2084867c12ee0c2ca47f",
"email": "[email protected]",
"fullName": "Irving Washington"
},
"paymentMethod": {
"_id": "64da2367d8d9771c02befbb2",
"method": "STRIPE",
"last4": "4242",
"brand": "visa"
}
}
]
}
}
Posting an Immediate Payment
Request
curl 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 500,
"note": "Deposit"
}
'
var myHeaders = new Headers();
myHeaders.append("accept", "application/json");
myHeaders.append("content-type", "application/json");
var raw = JSON.stringify({
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 500,
"note": "Deposit"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64da254383ad1500284bd0c0/payments',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'
{
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": true,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 500,
"note": "Deposit"
}
',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"paymentMethod": {
"method": "AMARYLLIS",
"saveForFutureUse": True,
"id": "64da2367d8d9771c02befbb2"
},
"amount": 500,
"note": "Deposit"
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/reservations/64da254383ad1500284bd0c0/payments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "64da254383ad1500284bd0c0",
"money": {
"payments": [
{
"paymentMethodStatus": "ACTIVE",
"isAuthorizationHold": false,
"status": "SUCCEEDED",
"_id": "64da267c83ad1500284bd104",
"chargebacksData": {
"chargebacks": []
},
"amount": 500,
"note": "Deposit",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"guestId": "64da2084867c12ee0c2ca47f",
"currency": "USD",
"attempts": [
{
"_id": "64da267d83ad1500284bd109",
"status": "SUCCEEDED",
"payload": {
"id": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"object": "payment_intent",
"amount": 50000,
"amount_capturable": 0,
"amount_received": 50000,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee_amount": 200,
"automatic_payment_methods": null,
"canceled_at": null,
"cancellation_reason": null,
"capture_method": "automatic",
"charges": {
"object": "list",
"data": [
{
"id": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"object": "charge",
"amount": 50000,
"amount_captured": 50000,
"amount_refunded": 0,
"application": "ca_7S8pfqtl3Am55FsbIOZcBbZivQqEDeUd",
"application_fee": "fee_1Nf0S9GfrrmRCKB1dolQDBpR",
"application_fee_amount": 200,
"balance_transaction": "txn_3Nf0S8GfrrmRCKB11uWFFji9",
"billing_details": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"email": null,
"name": "John Smith",
"phone": null
},
"calculated_statement_descriptor": "GUESTY BOOKING",
"captured": true,
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"destination": null,
"dispute": null,
"disputed": false,
"failure_balance_transaction": null,
"failure_code": null,
"failure_message": null,
"invoice": null,
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"on_behalf_of": null,
"order": null,
"outcome": {
"network_status": "approved_by_network",
"reason": null,
"risk_level": "normal",
"risk_score": 6,
"seller_message": "Payment complete.",
"type": "authorized"
},
"paid": true,
"payment_intent": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_details": {
"card": {
"brand": "visa",
"checks": {
"address_line1_check": "pass",
"address_postal_code_check": "pass",
"cvc_check": "pass"
},
"country": "US",
"ds_transaction_id": null,
"exp_month": 12,
"exp_year": 2026,
"fingerprint": "6i2ad2EHQpQJ8PeH",
"funding": "credit",
"installments": null,
"last4": "4242",
"mandate": null,
"moto": null,
"network": "visa",
"network_token": {
"used": false
},
"three_d_secure": null,
"wallet": null
},
"type": "card"
},
"receipt_email": null,
"receipt_number": null,
"receipt_url": "https://pay.stripe.com/receipts/payment/CAcaFwoVYWNjdF8xS2ZnTjRHZnJybVJDS0IxKP3M6KYGMgZvQW4a3jo6LBbT8xvMIgN5WV4QbH4rgdJw1K66qhPQKUU-PeqLw5GaFO_FgOJelXWlJsNI",
"refunded": false,
"refunds": {
"object": "list",
"data": [],
"has_more": false,
"total_count": 0,
"url": "/v1/charges/ch_3Nf0S8GfrrmRCKB1160cJBx4/refunds"
},
"review": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"source_transfer": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
}
],
"has_more": false,
"total_count": 1,
"url": "/v1/charges?payment_intent=pi_3Nf0S8GfrrmRCKB11SVF4dCI"
},
"client_secret": "pi_3Nf0S8GfrrmRCKB11SVF4dCI_secret_xrh2J2yBNN3IDaOcVQK2BwxZv",
"confirmation_method": "manual",
"created": 1692018300,
"currency": "usd",
"customer": "cus_ORu3dk6cGpYw0u",
"description": "r2B9jZYJK",
"invoice": null,
"last_payment_error": null,
"latest_charge": "ch_3Nf0S8GfrrmRCKB1160cJBx4",
"livemode": false,
"metadata": {
"OTA": "Manual",
"accountId": "5213a2d206112710005d96ff",
"confirmationCode": "r2B9jZYJK",
"guestName": "Irving Washington",
"listingId": "64da223eb3de7a002a462fcc",
"paymentId": "64da267c83ad1500284bd104",
"paymentMethodId": "64da2367d8d9771c02befbb2",
"reservationId": "64da254383ad1500284bd0c0"
},
"next_action": null,
"on_behalf_of": null,
"payment_method": "pm_1Nf0FOGfrrmRCKB1k7y7M57E",
"payment_method_options": {
"card": {
"installments": null,
"mandate_options": null,
"network": null,
"request_three_d_secure": "automatic"
}
},
"payment_method_types": [
"card"
],
"processing": null,
"receipt_email": null,
"review": null,
"setup_future_usage": null,
"shipping": {
"address": {
"city": "New York",
"country": "US",
"line1": "20 W 34th St",
"line2": null,
"postal_code": "10001",
"state": null
},
"carrier": null,
"name": "Irving Washington",
"phone": "12128765234",
"tracking_number": null
},
"source": null,
"statement_descriptor": "Guesty booking",
"statement_descriptor_suffix": null,
"status": "succeeded",
"transfer_data": null,
"transfer_group": null
},
"createdAt": "2023-08-14T13:05:01.910Z"
}
],
"shouldBePaidAt": "2023-08-14T13:05:00.263Z",
"paidAt": "2023-08-14T13:05:01.820Z",
"confirmationCode": "pi_3Nf0S8GfrrmRCKB11SVF4dCI",
"refunds": [],
"authorizationHoldCaptures": [],
"createdAt": "2023-08-14T13:05:01.910Z",
"receiptTargets": [],
"receiptId": 18801740,
"guest": {
"_id": "64da2084867c12ee0c2ca47f",
"email": "[email protected]",
"fullName": "Irving Washington"
},
"paymentMethod": {
"_id": "64da2367d8d9771c02befbb2",
"method": "STRIPE",
"last4": "4242",
"brand": "visa"
}
}
]
}
}
Note
If more than one payment is posted to the reservation, the response will include each payment object in its
payments
array.
Updated 11 months ago