Create a Reservation
How to create a reservation using the OAPI
Read First
The preferred and current reservation flow implemented in Guesty is the Reservation quote flow of our Booking Engine API (BEAPI). Only if you have an existing integration based on our legacy workflow should you rely on this. Still, the better option is to update your integration to use our BEAPI.
Booking Options
Reservations can be categorized into two primary categories:
- Booking request
- Instant book
Booking Request
A booking request represents a query from potential or returning guests. These are unconfirmed potential bookings.
- To create an inquiry with reserved dates, set the reservation status to
reserved
. - To create an inquiry without reserved dates, set the reservation status to
inquiry
.
Instant Book
This is a reservation that is confirmed in a single step. Simply set the reservation status to confirmed
when creating it.
Creating the Reservation
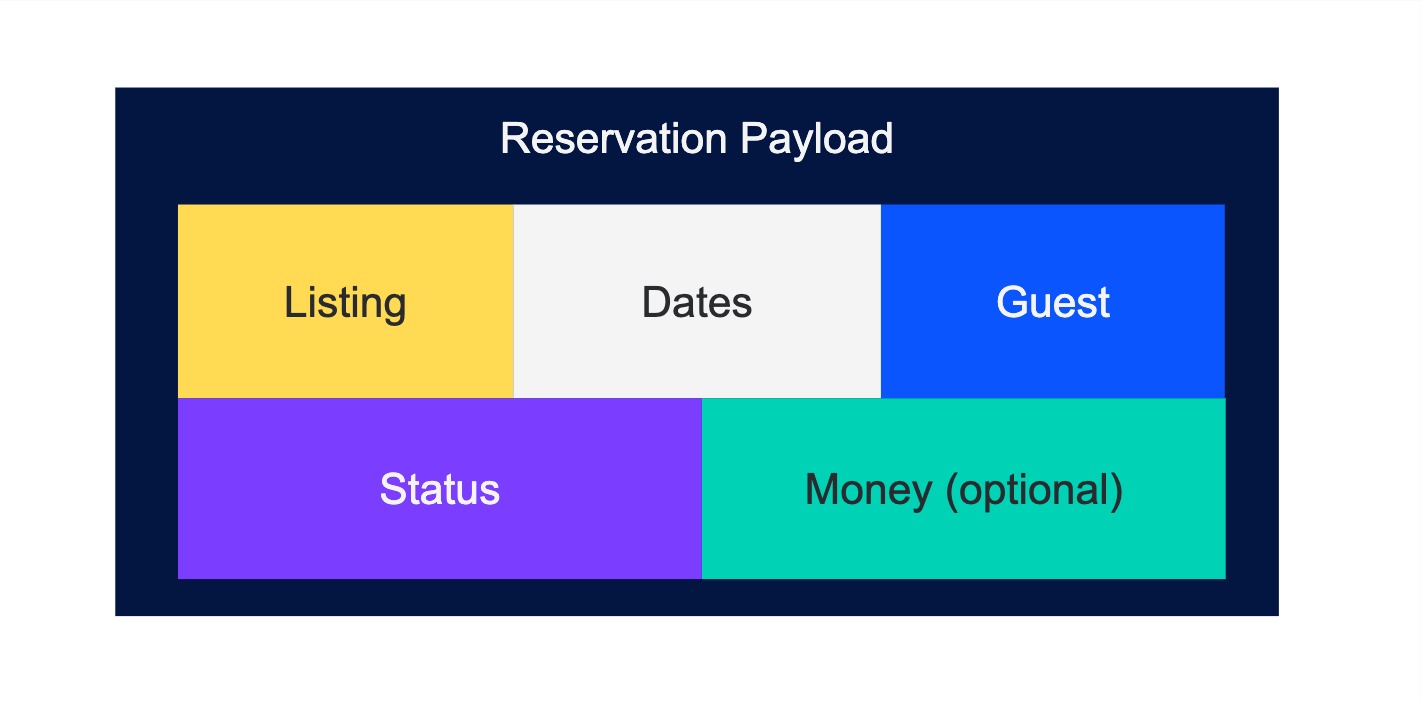
Reservation Payload
Available Endpoints
Method | Endpoint |
---|---|
POST | /reservations |
Key Parameters
Body Parameter | Type | Description | Required |
---|---|---|---|
listingId | string | The ID of the property being booked. | ✔️ |
checkInDateLocalized | string | The start date of the reservation according to the property's timezone. It should be written, YYYY-MM-DD . | ✔️ |
checkOutDateLocalized | string | The end date of the reservation according to the property's timezone. It should be written, YYYY-MM-DD . | ✔️ |
status | string | See this enumerations table for more information. | ✔️ |
money | object | The invoice items and currency. | |
guestId | string | The ID of the guest making the reservation. | ✔️ |
guest | object | When creating a new guest profile with the reservation. | ✔️ |
Money Object
Body Parameter | Type | Description | Required |
---|---|---|---|
fareAccommodation | number | Only if the amount must differ from the existing rate strategy. | |
fareCleaning | number | The cleaning fee. Specify it only if it differs from what is set on the listing. | |
invoiceItems | [object] | An array of other fees. For including additional fees on the reservation (See the Invoice Items table below). | |
currency | string | The currency of the invoice. A list of supported values can be found [here]. Only specify if it differed from the currency set for the listing. |
Invoice Items
You may add additional fees or taxes from those you've configured on your Guesty account (just make sure none of them apply automatically, or you will have duplicates) or create your own fee with the relevant normalType
.
Body Parameter | Type | Description | Required |
---|---|---|---|
title | string | The name of the fee. | ✔️ |
amount | number | The amount to charge. | ✔️ |
normalType | string | The fee category. See this table of enums. | ✔️ |
secondIdentifier | string | An additional identifier. Normally, a variant of the fee name and time. See this table of enums for predefined identifiers. | ✔️ |
description | string | Add an additional description to add further context to the fee. |
Override Parameters
These parameters should be used sparingly with care and caution. If you've set up your properties well, you'll find that you may never need to employ them.
Overbookings
You are responsible for any overbooking incurred as a result of the use of these parameters.
Body Parameter | Type | Description | Required |
---|---|---|---|
ignoreCalendar | boolean | Default: false . Set to true to override any calendar blocks (including other reservations). | |
ignoreTerms | boolean | Default: false . Set to true if you wish to overlook the listing terms such as max occupancy, min. nights, etc. |
Reservation Statuses
Status | Description |
---|---|
inquiry | A question from a potential guest or returning one. |
declined | This status is used when the host rejects an inquiry. |
expired | An expired inquiry, request, or quote. |
canceled | A canceled reservation (previously confirmed) |
closed | A booking request that wasn't or isn't confirmed |
reserved | A booking request. Calendar dates are reserved. |
confirmed | A confirmed reservation. |
Example
Request
This request follows the flow where the guest was created first. Only the guestId
is included.
curl 'https://open-api.guesty.com/v1/reservations' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '{
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-01",
"checkOutDateLocalized": "2023-09-10",
"status": "reserved",
"guestId": "64c7afe8a1237cba15116c95"
}'
var myHeaders = new Headers();
myHeaders.append("accept", "application/json");
myHeaders.append("content-type", "application/json");
var raw = JSON.stringify({
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-01",
"checkOutDateLocalized": "2023-09-10",
"status": "reserved",
"guestId": "64c7afe8a1237cba15116c95"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-01",
"checkOutDateLocalized": "2023-09-10",
"status": "reserved",
"guestId": "64c7afe8a1237cba15116c95"
}',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-01",
"checkOutDateLocalized": "2023-09-10",
"status": "reserved",
"guestId": "64c7afe8a1237cba15116c95"
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/reservations", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"integration": {
"limitations": {
"availableStatuses": []
},
"bookingCom": {
"invalidCreditCards": [],
"reports": []
},
"_id": "5b9a25fd3bfa6d01b15a0b14",
"platform": "manual",
"object": {
"airbnb": {
"ignored": {
"listings": [],
"reservations": []
},
"importCalendars": true
},
"rentalsUnited": {
"ignored": {
"listings": [],
"reservations": []
},
"cleaningFeeIncludedInNightlyPrice": {
"agoda": true,
"bookingCom": false,
"canadaStays": true,
"despegar": true,
"expedia": true,
"homeAway": true,
"hostelworld": true
}
},
"bookingCom": {
"ignored": {
"listings": [],
"reservations": []
}
},
"homeAway": {
"ignored": {
"listings": [],
"reservations": []
}
},
"homeaway2": {
"ignored": {
"listings": []
}
},
"agoda": {
"ignored": {
"listings": [],
"reservations": []
}
},
"tripAdvisor": {
"ignored": {
"listings": [],
"reservations": []
}
},
"_id": "5b9a25fd3bfa6d01b15a0b14",
"platform": "manual",
"incomingEmail": "[email protected]",
"nickname": "demobnb_oe6yqvg",
"createAt": "2018-09-13T08:55:25.034Z",
"active": true,
"createdAt": "2018-09-13T08:55:25.000Z"
}
},
"review": {
"shouldReview": true
},
"atTimeOfConfirmation": {
"snapshotCreated": false,
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"taxes": []
},
"earlyCheckIn": {
"blockDay": false
},
"lateCheckOut": {
"blockDay": false
},
"flag": false,
"accountingEnabled": true,
"isBMApplied": false,
"confirmedPreBookings": [],
"sourceMeta": {
"guestyUrl": null,
"customUrl": null,
"businessName": null
},
"pulledByDailySync": false,
"manuallyCreated": false,
"_id": "64c91cb2a097ec00417a8575",
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-01",
"checkOutDateLocalized": "2023-09-10",
"status": "reserved",
"guestId": "64c7afe8a1237cba15116c95",
"accountId": "5b852676354b34003f0a55eb",
"guestsCount": 1,
"money": {
"settingsSnapshot": {
"currency": {
"rate": 1
},
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"additionalFees": [],
"snapshotCreated": false,
"taxes": []
},
"channelCommissionRules": null,
"paymentProviderIds": [
"5fcdf594f6967b002c58bee6"
],
"altered": false,
"_id": "64c91cb3a27d63003dc4784d",
"version": 1,
"currency": "AUD",
"fareAccommodation": 900,
"fareAccommodationAdjusted": 900,
"fareCleaning": 0,
"invoiceItems": [
{
"_id": "64c91cb3a27d63003dc4784e",
"title": "Accommodation fare",
"amount": 900,
"currency": "AUD",
"type": "ACCOMMODATION_FARE",
"isLocked": true,
"normalType": "AF"
},
{
"_id": "64c91cb3a27d63003dc4784f",
"title": "VAT",
"amount": 180,
"currency": "AUD",
"isLocked": true,
"isTax": true,
"type": "TAX",
"metadata": {
"calculated": true
},
"normalType": "VAT"
}
],
"fareAccommodationAdjustment": 0,
"fareAccommodationDiscount": 0,
"hostServiceFee": 0,
"hostServiceFeeTax": 0,
"hostServiceFeeIncTax": 0,
"totalFees": 0,
"subTotalPrice": 900,
"hostPayout": 1080,
"hostPayoutUsd": 714.86,
"totalTaxes": 180,
"useAccountRevenueShare": true,
"netIncomeFormula": "host_payout",
"netIncome": 1080,
"commissionFormula": "0",
"commission": 0,
"commissionTaxPercentage": 0,
"commissionTax": 0,
"commissionIncTax": 0,
"ownerRevenueFormula": "net_income",
"ownerRevenue": 1080,
"platform": "manual",
"reservationId": "64c91cb2a097ec00417a8575",
"createdAt": "2023-08-01T14:54:43.429Z",
"updatedAt": "2023-08-01T14:54:43.429Z",
"payments": [],
"balanceDue": 1080,
"isFullyPaid": false,
"paymentsDue": 0,
"totalRefunded": 0,
"totalPaid": 0,
"autoPaymentsPolicy": []
},
"source": "Manual",
"confirmationCode": "jY5RZ8WVP",
"mtl": {
"assigned": false,
"_id": "64c91cb3a097ec00417a857b"
},
"isReturningGuest": false,
"checkIn": "2023-09-01T05:00:00.000Z",
"checkOut": "2023-09-10T00:00:00.000Z",
"nightsCount": 9,
"additionalFeesAtCreation": [],
"guestyFeeDetails": {
"commission": 0,
"planItems": [],
"feeMinimum": 0,
"fee": 0,
"feeUsd": 0,
"isMinimumFee": false,
"isMaximumFee": false
},
"createdAt": "2023-08-01T14:54:43.511Z",
"pendingTasks": [],
"customFields": [],
"lastUpdatedAt": "2023-08-01T14:54:43.516Z",
"guest": {
"airbnb": {
"id": "1180340812902"
},
"airbnb2": {
"index": "index",
"id": "1180340821319"
},
"policy": {
"marketing": {
"isAccepted": false
},
"termsAndConditions": {
"isAccepted": false
}
},
"verifications": [],
"emails": [
"[email protected]"
],
"phones": [
"12128765234"
],
"communicationMethods": [
"email",
"sms"
],
"interests": [],
"allergies": [],
"dietaryPreferences": [],
"tags": [],
"_id": "64c7afe8a1237cba15116c95",
"createdAt": "2023-07-31T12:43:33.218Z",
"paymentProvidersCustomers": [],
"nationality": "us",
"isOtaModified": false,
"otaLinks": [],
"pronouns": "he/him/his",
"gender": "male",
"preferredLanguage": "en",
"phone": "12128765234",
"email": "[email protected]",
"hometown": "New York",
"fullName": "Irving Washington",
"lastName": "Washington",
"firstName": "Irving"
},
"listing": {
"address": {
"full": "77 King St, Sydney NSW 2000, Australia",
"lng": 151.2066116,
"lat": -33.8689358,
"street": "King Street 77",
"city": "Sydney",
"country": "Australia",
"zipcode": "2000",
"state": "New South Wales"
},
"cleaningStatus": {
"value": "dirty",
"updatedBy": "Guesty automation",
"updatedAt": "2023-04-04T05:46:27.378Z"
},
"picture": {
"thumbnail": "https://assets.guesty.com/image/upload/c_fit,h_200/v1659660646/production/5b852676354b34003f0a55eb/wd2scokycc6pbbyrnty2.jpg"
},
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "AUD",
"basePrice": 100
},
"type": "SINGLE",
"tags": [
"shan"
],
"owners": [],
"amenities": [
"Air conditioning",
"Elevator",
"Refrigerator",
"Stove",
"TV"
],
"amenitiesNotIncluded": [],
"useAccountRevenueShare": true,
"netIncomeFormula": "host_payout",
"commissionFormula": "net_income",
"ownerRevenueFormula": "net_income - pm_commission",
"useAccountAdditionalFees": true,
"taxes": [],
"useAccountTaxes": true,
"useAccountMarkups": true,
"active": true,
"preBooking": [],
"_id": "62ec6975134d780032b62221",
"accommodates": 2,
"bathrooms": 1,
"defaultCheckInTime": "15:00",
"defaultCheckOutTime": "10:00",
"propertyType": "Apartment",
"roomType": "Entire home/apt",
"timezone": "Australia/Sydney",
"nickname": "Test_Listing_Shan",
"title": "Test Listing Shans",
"isListed": true,
"createdAt": "2022-08-05T00:51:01.490Z",
"lastUpdatedAt": "2023-07-30T11:30:29.965Z",
"integrations": [],
"listingRooms": [
{
"_id": "64c649d5a42142003f078e79",
"roomNumber": 0,
"beds": [
{
"_id": "64c649d5a42142003f078e7a",
"type": "SOFA_BED",
"quantity": 1
}
]
},
{
"_id": "64c649d5a42142003f078e7b",
"roomNumber": 1,
"beds": [
{
"_id": "64c649d5a42142003f078e7c",
"type": "KING_BED",
"quantity": 1
}
]
}
],
"customFields": [],
"importedAt": "2022-08-05T00:51:01.491Z",
"occupancyStats": [],
"promotions": [
"62ec68ee9d862b00333379b8"
],
"bedrooms": 1,
"beds": 2
},
"__v": 0,
"id": "64c91cb2a097ec00417a8575"
}
Request
This request includes the guest object for creating a new guest profile with the reservation. If the guest does exist, that profile will be linked with the reservation and updated with any new or altered information.
curl 'https://open-api.guesty.com/v1/reservations' \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data-raw '{
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-12",
"checkOutDateLocalized": "2023-09-20",
"status": "reserved",
"guest": {
"firstName": "Ernest",
"lastName": "Hemingway",
"email": "[email protected]",
"phone": "+123456789",
"nationality": "us",
"gender": "male",
"preferredLanguage": "en"
}
}'
var myHeaders = new Headers();
myHeaders.append("accept", "application/json");
myHeaders.append("content-type", "application/json");
var raw = JSON.stringify({
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-12",
"checkOutDateLocalized": "2023-09-20",
"status": "reserved",
"guest": {
"firstName": "Ernest",
"lastName": "Hemingway",
"email": "[email protected]",
"phone": "+123456789",
"nationality": "us",
"gender": "male",
"preferredLanguage": "en"
}
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-12",
"checkOutDateLocalized": "2023-09-20",
"status": "reserved",
"guest": {
"firstName": "Ernest",
"lastName": "Hemingway",
"email": "[email protected]",
"phone": "+123456789",
"nationality": "us",
"gender": "male",
"preferredLanguage": "en"
}
}',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'content-type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
import json
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = json.dumps({
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-12",
"checkOutDateLocalized": "2023-09-20",
"status": "reserved",
"guest": {
"firstName": "Ernest",
"lastName": "Hemingway",
"email": "[email protected]",
"phone": "+123456789",
"nationality": "us",
"gender": "male",
"preferredLanguage": "en"
}
})
headers = {
'accept': 'application/json',
'content-type': 'application/json'
}
conn.request("POST", "/v1/reservations", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"integration": {
"limitations": {
"availableStatuses": []
},
"bookingCom": {
"invalidCreditCards": [],
"reports": []
},
"_id": "5b9a25fd3bfa6d01b15a0b14",
"platform": "manual",
"object": {
"airbnb": {
"ignored": {
"listings": [],
"reservations": []
},
"importCalendars": true
},
"rentalsUnited": {
"ignored": {
"listings": [],
"reservations": []
},
"cleaningFeeIncludedInNightlyPrice": {
"agoda": true,
"bookingCom": false,
"canadaStays": true,
"despegar": true,
"expedia": true,
"homeAway": true,
"hostelworld": true
}
},
"bookingCom": {
"ignored": {
"listings": [],
"reservations": []
}
},
"homeAway": {
"ignored": {
"listings": [],
"reservations": []
}
},
"homeaway2": {
"ignored": {
"listings": []
}
},
"agoda": {
"ignored": {
"listings": [],
"reservations": []
}
},
"tripAdvisor": {
"ignored": {
"listings": [],
"reservations": []
}
},
"_id": "5b9a25fd3bfa6d01b15a0b14",
"platform": "manual",
"incomingEmail": "[email protected]",
"nickname": "demobnb_oe6yqvg",
"createAt": "2018-09-13T08:55:25.034Z",
"active": true,
"createdAt": "2018-09-13T08:55:25.000Z"
}
},
"review": {
"shouldReview": true
},
"atTimeOfConfirmation": {
"snapshotCreated": false,
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"taxes": []
},
"earlyCheckIn": {
"blockDay": false
},
"lateCheckOut": {
"blockDay": false
},
"flag": false,
"accountingEnabled": true,
"isBMApplied": false,
"confirmedPreBookings": [],
"sourceMeta": {
"guestyUrl": null,
"customUrl": null,
"businessName": null
},
"pulledByDailySync": false,
"manuallyCreated": false,
"_id": "64c9213ba097ec00417a9911",
"listingId": "62ec6975134d780032b62221",
"checkInDateLocalized": "2023-09-12",
"checkOutDateLocalized": "2023-09-20",
"status": "reserved",
"guest": {
"airbnb": {
"id": "11033544172381"
},
"airbnb2": {
"index": "index",
"id": "11033544250497"
},
"policy": {
"marketing": {
"isAccepted": false,
"dateOfAcceptance": null
},
"termsAndConditions": {
"isAccepted": false
}
},
"verifications": [],
"emails": [
"[email protected]"
],
"phones": [
"123456789"
],
"communicationMethods": [
"email",
"sms"
],
"interests": [],
"allergies": [],
"dietaryPreferences": [],
"tags": [],
"_id": "64c9213aa097ec00417a9910",
"firstName": "Ernest",
"lastName": "Hemingway",
"email": "[email protected]",
"phone": "123456789",
"nationality": "us",
"gender": "male",
"preferredLanguage": "en",
"paymentProvidersCustomers": [],
"fullName": "Ernest Hemingway"
},
"accountId": "5b852676354b34003f0a55eb",
"guestId": "64c9213aa097ec00417a9910",
"guestsCount": 1,
"money": {
"settingsSnapshot": {
"currency": {
"rate": 1
},
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"additionalFees": [],
"snapshotCreated": false,
"taxes": []
},
"channelCommissionRules": null,
"paymentProviderIds": [
"5fcdf594f6967b002c58bee6"
],
"altered": false,
"_id": "64c9213b686b7700357e8f25",
"version": 1,
"currency": "AUD",
"fareAccommodation": 800,
"fareAccommodationAdjusted": 800,
"fareCleaning": 0,
"invoiceItems": [
{
"_id": "64c9213b686b7700357e8f26",
"title": "Accommodation fare",
"amount": 800,
"currency": "AUD",
"type": "ACCOMMODATION_FARE",
"isLocked": true,
"normalType": "AF"
},
{
"_id": "64c9213b686b7700357e8f27",
"title": "VAT",
"amount": 160,
"currency": "AUD",
"isLocked": true,
"isTax": true,
"type": "TAX",
"metadata": {
"calculated": true
},
"normalType": "VAT"
}
],
"fareAccommodationAdjustment": 0,
"fareAccommodationDiscount": 0,
"hostServiceFee": 0,
"hostServiceFeeTax": 0,
"hostServiceFeeIncTax": 0,
"totalFees": 0,
"subTotalPrice": 800,
"hostPayout": 960,
"hostPayoutUsd": 635.29,
"totalTaxes": 160,
"useAccountRevenueShare": true,
"netIncomeFormula": "host_payout",
"netIncome": 960,
"commissionFormula": "0",
"commission": 0,
"commissionTaxPercentage": 0,
"commissionTax": 0,
"commissionIncTax": 0,
"ownerRevenueFormula": "net_income",
"ownerRevenue": 960,
"platform": "manual",
"reservationId": "64c9213ba097ec00417a9911",
"createdAt": "2023-08-01T15:14:03.975Z",
"updatedAt": "2023-08-01T15:14:03.975Z",
"payments": [],
"balanceDue": 960,
"isFullyPaid": false,
"paymentsDue": 0,
"totalRefunded": 0,
"totalPaid": 0,
"autoPaymentsPolicy": []
},
"source": "Manual",
"confirmationCode": "r2Bk86Yj4",
"mtl": {
"assigned": false,
"_id": "64c9213ca097ec00417a9917"
},
"isReturningGuest": false,
"checkIn": "2023-09-12T05:00:00.000Z",
"checkOut": "2023-09-20T00:00:00.000Z",
"nightsCount": 8,
"additionalFeesAtCreation": [],
"guestyFeeDetails": {
"commission": 0,
"planItems": [],
"feeMinimum": 0,
"fee": 0,
"feeUsd": 0,
"isMinimumFee": false,
"isMaximumFee": false
},
"createdAt": "2023-08-01T15:14:04.049Z",
"pendingTasks": [],
"customFields": [],
"lastUpdatedAt": "2023-08-01T15:14:04.054Z",
"listing": {
"address": {
"full": "77 King St, Sydney NSW 2000, Australia",
"lng": 151.2066116,
"lat": -33.8689358,
"street": "King Street 77",
"city": "Sydney",
"country": "Australia",
"zipcode": "2000",
"state": "New South Wales"
},
"cleaningStatus": {
"value": "dirty",
"updatedBy": "Guesty automation",
"updatedAt": "2023-04-04T05:46:27.378Z"
},
"picture": {
"thumbnail": "https://assets.guesty.com/image/upload/c_fit,h_200/v1659660646/production/5b852676354b34003f0a55eb/wd2scokycc6pbbyrnty2.jpg"
},
"terms": {
"minNights": 1,
"maxNights": 45
},
"prices": {
"monthlyPriceFactor": 1,
"weeklyPriceFactor": 1,
"currency": "AUD",
"basePrice": 100
},
"type": "SINGLE",
"tags": [
"shan"
],
"owners": [],
"amenities": [
"Air conditioning",
"Elevator",
"Refrigerator",
"Stove",
"TV"
],
"amenitiesNotIncluded": [],
"useAccountRevenueShare": true,
"netIncomeFormula": "host_payout",
"commissionFormula": "net_income",
"ownerRevenueFormula": "net_income - pm_commission",
"useAccountAdditionalFees": true,
"taxes": [],
"useAccountTaxes": true,
"useAccountMarkups": true,
"active": true,
"preBooking": [],
"_id": "62ec6975134d780032b62221",
"accommodates": 2,
"bathrooms": 1,
"defaultCheckInTime": "15:00",
"defaultCheckOutTime": "10:00",
"propertyType": "Apartment",
"roomType": "Entire home/apt",
"timezone": "Australia/Sydney",
"nickname": "Test_Listing_Shan",
"title": "Test Listing Shans",
"isListed": true,
"createdAt": "2022-08-05T00:51:01.490Z",
"lastUpdatedAt": "2023-07-30T11:30:29.965Z",
"integrations": [],
"listingRooms": [
{
"_id": "64c649d5a42142003f078e79",
"roomNumber": 0,
"beds": [
{
"_id": "64c649d5a42142003f078e7a",
"type": "SOFA_BED",
"quantity": 1
}
]
},
{
"_id": "64c649d5a42142003f078e7b",
"roomNumber": 1,
"beds": [
{
"_id": "64c649d5a42142003f078e7c",
"type": "KING_BED",
"quantity": 1
}
]
}
],
"customFields": [],
"importedAt": "2022-08-05T00:51:01.491Z",
"occupancyStats": [],
"promotions": [
"62ec68ee9d862b00333379b8"
],
"bedrooms": 1,
"beds": 2
},
"__v": 0,
"id": "64c9213ba097ec00417a9911"
}
Alterations
When manipulating reservations created with BEAPI or via the Open API reservations endpoints, please allow up to 60 seconds between requests to allow each to complete and achieve the expected outcome.
Troubleshooting
Status 500 Internal Server Error: Integration not found
If you have not created or received direct bookings on your Guesty account. Please activate the booking engine by creating a test reservation in the UI. API reservation requests require this activation to work.
Updated 10 months ago