Recording an External Guest Payment/Refund
Use this guide to understand how to record external payments/refunds on a reservation.
Overview
This document details the process for recording external guest payments and refunds using a payment method not integrated with Guesty.
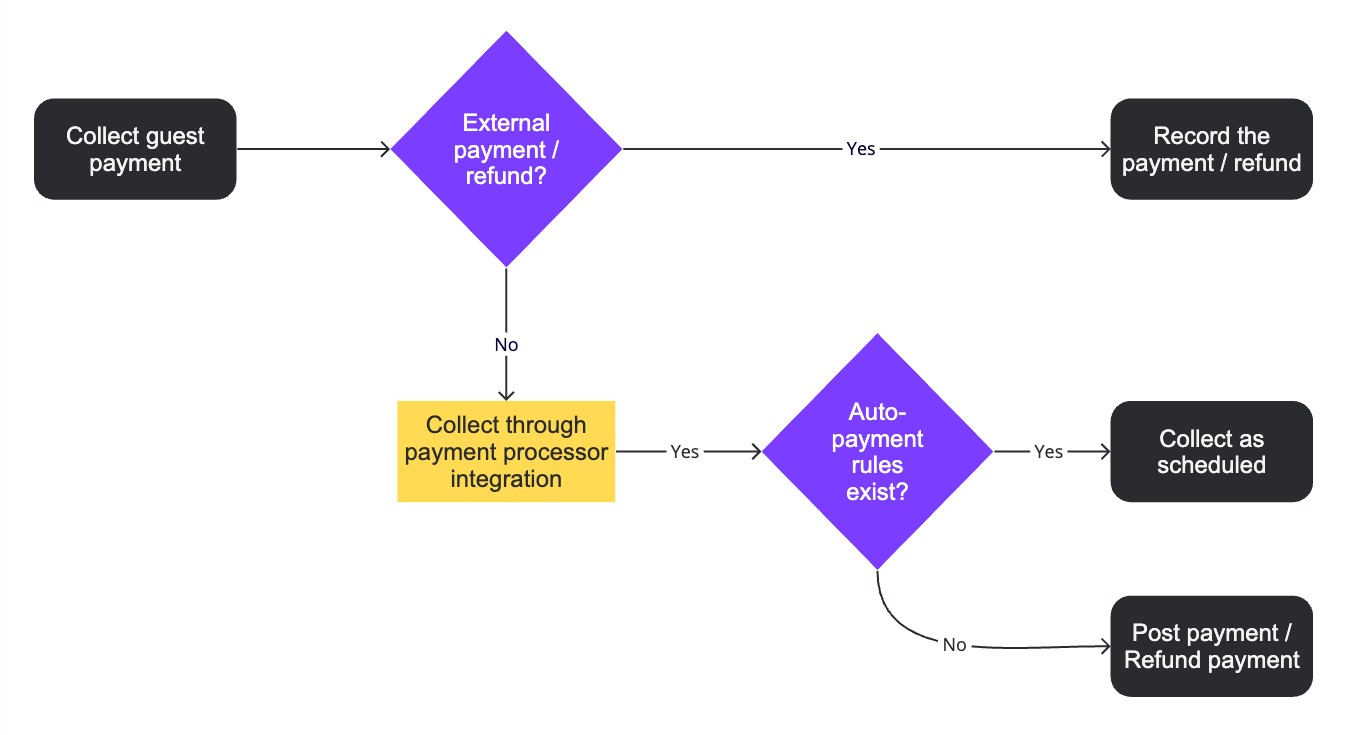
Recording an External Payment Flow
Response Payload
The response will return all the payment objects posted to the reservation. Identify the most recent payment object to confirm its success.
Recording a Payment
Available Endpoints
Method | Endpoint |
---|---|
POST | /reservations/{id}/payments |
Key Parameters
Path Parameter | Description | Data Type | Required |
---|---|---|---|
_id | The reservation ID. | string | ✓ |
Body Parameter | Description | Data Type | Required |
---|---|---|---|
paymentMethod | The payment method object. | object | ✓ |
- method | Enter the payment method. See Enums. | string | ✓ |
-saveForFutureUse | true or false | boolean | |
amount | The amount to charge the guest. | float | ✓ |
shouldBePaidAt | Enter the date in YYYY-MM-DD format to schedule the payment. Refrain from using this when recording payments. See the callout below. | date | |
paidAt | Enter the date in YYYY-MM-DD format to process the payment with a past date. | date | |
note | Describe the payment. | string |
Scheduling Recorded Payments
Recording a payment with a future date is strongly discouraged and can lead to the payment failing to be counted at the allotted time. In addition, if it is a
CASH
payment and the reservation has a payment method, the record may become an actual transaction using your integrated payment provider solution.To attain the best outcome, we recommend you omit
paidAt
andshouldBePaidAt
from your call to record the payment with the current date and time or includepaidAt
to record it with a past date and time.
Example
Request
curl 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments' \
--data '{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"amount": 1000,
"paidAt": "2023-08-24T16:00:00.000Z",
"note": "First installment"
}'
var raw = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\n },\n \"amount\": 1000,\n \"paidAt\": \"2023-08-24T16:00:00.000Z\",\n \"note\": \"First installment\"\n}";
var requestOptions = {
method: 'POST',
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"amount": 1000,
"paidAt": "2023-08-24T16:00:00.000Z",
"note": "First installment"
}',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\n },\n \"amount\": 1000,\n \"paidAt\": \"2023-08-24T16:00:00.000Z\",\n \"note\": \"First installment\"\n}"
headers = {}
conn.request("POST", "/v1/reservations/64873771ec565e0042006d13/payments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id":"64873771ec565e0042006d13",
"money":{
"payments":[
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2a",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704f",
"shouldBePaidAt":"2023-06-12T15:19:13.699Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.385Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2b",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704e",
"shouldBePaidAt":"2023-09-02T22:00:00.000Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.386Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64e75950c0173f004f71f929",
"chargebacksData":{
"chargebacks":[
]
},
"amount":1000,
"paidAt":"2023-08-24T16:00:00.000Z",
"note":"First installment",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:00:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:21:20.068Z",
"receiptTargets":[
],
"receiptId":26367986,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
}
]
}
}
Recording a Refund
To record a refund, enter a negative value as the amount in the request, which is the same as the one for payment.
Example
Request
curl 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments' \
--data '{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"amount": -200,
"paidAt": "2023-08-24T16:30:00.000Z",
"note": "Early payment discount"
}'
var raw = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\n },\n \"amount\": -200,\n \"paidAt\": \"2023-08-24T16:30:00.000Z\",\n \"note\": \"Early payment discount\"\n}";
var requestOptions = {
method: 'POST',
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"amount": -200,
"paidAt": "2023-08-24T16:30:00.000Z",
"note": "Early payment discount"
}',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\n },\n \"amount\": -200,\n \"paidAt\": \"2023-08-24T16:30:00.000Z\",\n \"note\": \"Early payment discount\"\n}"
headers = {}
conn.request("POST", "/v1/reservations/64873771ec565e0042006d13/payments", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id":"64873771ec565e0042006d13",
"money":{
"payments":[
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2a",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704f",
"shouldBePaidAt":"2023-06-12T15:19:13.699Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.385Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2b",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704e",
"shouldBePaidAt":"2023-09-02T22:00:00.000Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.386Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64e75950c0173f004f71f929",
"chargebacksData":{
"chargebacks":[
]
},
"amount":1000,
"paidAt":"2023-08-24T16:00:00.000Z",
"note":"First installment",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:00:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:21:20.068Z",
"receiptTargets":[
],
"receiptId":26367986,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64e75bdda3f13a004393e116",
"chargebacksData":{
"chargebacks":[
]
},
"amount":-200,
"paidAt":"2023-08-24T16:30:00.000Z",
"note":"Early payment discount",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:30:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:32:13.270Z",
"receiptTargets":[
],
"receiptId":26368208,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
}
]
}
}
Editing a Recorded Payment/Refund
Available Endpoints
Method | Endpoint |
---|---|
POST | /reservations/{id}/payments |
PUT | /reservations/{id}/payments/{paymentId} |
1. Retrieve the Payment ID
Follow the instructions in the Refunding a Guest Payment guide.
2. Edit the Payment
To alter a payment for a reservation, please refer to the corresponding document that outlines the editable fields. In the following examples, the $200 refund is canceled, and the recorded payment is adjusted to $800.
Request
curl --request PUT 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments/64e75bdda3f13a004393e116' \
--data '{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"status": "CANCELLED"
}'
Response
{
"_id":"64873771ec565e0042006d13",
"money":{
"payments":[
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64873772ec565e0042006d2a",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704f",
"shouldBePaidAt":"2023-08-24T13:42:30.757Z",
"currency":"USD",
"amount":200,
"paymentMethodId":"58a1932b85e33bd55ec5c8c5",
"refunds":[
{
"_id":"64e75e69710ffd0035d931b9",
"amount":200,
"status":"SUCCEEDED",
"createdAt":"2023-08-24T13:43:05.595Z"
}
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.385Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"paidAt":"2023-08-24T13:42:30.757Z",
"receiptId":26368271,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"58a1932b85e33bd55ec5c8c5",
"method":"ECHECK"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2b",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704e",
"shouldBePaidAt":"2023-09-02T22:00:00.000Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.386Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"CANCELLED",
"_id":"64e75950c0173f004f71f929",
"chargebacksData":{
"chargebacks":[
]
},
"amount":1000,
"paidAt":"2023-08-24T16:00:00.000Z",
"note":"First installment",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:00:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:21:20.068Z",
"receiptTargets":[
],
"receiptId":26367986,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"CANCELLED",
"_id":"64e75bdda3f13a004393e116",
"chargebacksData":{
"chargebacks":[
]
},
"amount":-200,
"paidAt":"2023-08-24T16:30:00.000Z",
"note":"Early payment discount",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:30:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:32:13.270Z",
"receiptTargets":[
],
"receiptId":26368208,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
}
]
}
}
Request
curl --request PUT 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments/64e75950c0173f004f71f929' \
--data '{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"status": "SUCCEEDED",
"amount": 800
}'
var raw = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\ },\n \"status\": \"SUCCEEDED\",\n \"amount\": 800\n}";
var requestOptions = {
method: 'PUT',
body: raw,
redirect: 'manual'
};
fetch("https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments/64e75950c0173f004f71f929", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://open-api.guesty.com/v1/reservations/64873771ec565e0042006d13/payments/64e75950c0173f004f71f929',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS =>'{
"paymentMethod": {
"method": "BANK_TRANSFER"
},
"status": "SUCCEEDED",
"amount": 800
}',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("open-api.guesty.com")
payload = "{\n \"paymentMethod\": {\n \"method\": \"BANK_TRANSFER\",\n },\n \"status\": \"SUCCEEDED\",\n \"amount\": 800\n}"
headers = {}
conn.request("PUT", "/v1/reservations/64873771ec565e0042006d13/payments/64e75950c0173f004f71f929", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id":"64873771ec565e0042006d13",
"money":{
"payments":[
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64873772ec565e0042006d2a",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704f",
"shouldBePaidAt":"2023-08-24T13:42:30.757Z",
"currency":"USD",
"amount":200,
"paymentMethodId":"58a1932b85e33bd55ec5c8c5",
"refunds":[
{
"_id":"64e75e69710ffd0035d931b9",
"amount":200,
"status":"SUCCEEDED",
"createdAt":"2023-08-24T13:43:05.595Z"
}
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.385Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"paidAt":"2023-08-24T13:42:30.757Z",
"receiptId":26368271,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"58a1932b85e33bd55ec5c8c5",
"method":"ECHECK"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"PENDING",
"_id":"64873772ec565e0042006d2b",
"chargebacksData":{
"chargebacks":[
]
},
"policyItemId":"6384b34fee696c0041f2704e",
"shouldBePaidAt":"2023-09-02T22:00:00.000Z",
"currency":"USD",
"amount":1096.15,
"paymentMethodId":"589894a91d756b9c47ce1e87",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-06-12T15:19:14.386Z",
"attempts":[
],
"receiptTargets":[
],
"guestId":"6304135d6409db0035df92ea",
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"589894a91d756b9c47ce1e87",
"method":"CASH"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"SUCCEEDED",
"_id":"64e75950c0173f004f71f929",
"chargebacksData":{
"chargebacks":[
]
},
"amount":800,
"paidAt":"2023-08-24T16:00:00.000Z",
"note":"First installment",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:00:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:21:20.068Z",
"receiptTargets":[
],
"receiptId":26367986,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
},
{
"paymentMethodStatus":"ACTIVE",
"isAuthorizationHold":false,
"status":"CANCELLED",
"_id":"64e75bdda3f13a004393e116",
"chargebacksData":{
"chargebacks":[
]
},
"amount":-200,
"paidAt":"2023-08-24T16:30:00.000Z",
"note":"Early payment discount",
"paymentMethodId":"5dee4ebd32acdf7051cd6ed6",
"guestId":"6304135d6409db0035df92ea",
"currency":"USD",
"attempts":[
],
"shouldBePaidAt":"2023-08-24T16:30:00.000Z",
"refunds":[
],
"authorizationHoldCaptures":[
],
"createdAt":"2023-08-24T13:32:13.270Z",
"receiptTargets":[
],
"receiptId":26368208,
"guest":{
"_id":"6304135d6409db0035df92ea",
"email":"[email protected]",
"fullName":"Roger Rabbit"
},
"paymentMethod":{
"_id":"5dee4ebd32acdf7051cd6ed6",
"method":"BANK_TRANSFER"
}
}
]
}
}
Updated 24 days ago